|
ArduinoMega2560 |
x 1 | |
|
HuskylensDF Robot
|
x 1 | |
![]() |
servomotor |
x 1 | |
|
wheels kitKEYESTUDIO
|
x 1 | |
|
ultrasonic sensorELEGOO
|
x 1 | |
|
jumper wiregeneral
|
x 1 | |
![]() |
BBC MICRO:BIT |
x 1 | |
![]() |
PLA Filament |
x 1 | |
|
eletronic components (see bom file) |
x 1 |
![]() |
3D Printer (generic) |
|
![]() |
Ultimaker CURA |
|
![]() |
Autodesk Fusion 360Autodesk
|
|
![]() |
makecode microbit |
|
![]() |
Hot glue gun |
A robomaster-base robot
I had the idea for this robot some years ago, when I saw for the first time robomaster university, a competition where robots fight each other. The robot has got a four-wheel tank drive, a blaster, a gripper and a bunch of sensors like a huskylens AI, used to process auto targeting algorithms. It runs on an Arduino Mega and a Micro:bit, connected by serial communication. It also used a shield (files in description), for simplified connections between all the components. For this presentation I will split all the structure in small logic blocks, as followed:
- Control system (Arduino and Micro:bit);
- Actuators system (gripper and blaster);
- Sensors system (Huskylens and other smaller sensors)
CONTROL SYSTEM
- Overall view
It is formed by an Arduino Mega and a Micro:bit. The Arduino is responsible for collecting all the data sended by the sensor system and Micro:bit and elaborate inputs for the actuators system. The Micro:bit carries out more outputting functions, like transmitting data to others Micro:bit with various parameters (compass position, accelerometer data, minus data) or use its 5x5 LED matrix to send intuitive messages (like setting mode). It is connected with the Arduino with serial communication, and they also share the same +5V power supply.
- Code
There are two programs: one for the Arduino, and another for the Micro:bit. The first one is used for setting robots mode (more about later) and for inputting the majorities of controlling commands (a small part is left at the micro:bit). The code is written with a switch-state logic that guarantees a wide range of algorithms without significant lags or bugs. The most important modes are 1, 2 and 3. Other modes are only for test uses and don’t make significant changes on the robot behaviour. The mode 1 is the “standard mode”, where the robot has got most of the functions available. Mode 2 and 3 activate different algorithms used for tracking. Mode 2 is a normal “follow me” algorithm that uses coordinates sent by Huskylens for following a target. You can find the code in the “husk.h” section under the voice “mode 2”. Algorithm used for mode 3 is the most interesting part of the entire code. It uses some basic trigonometry to evaluate an angle for the blaster. As for mode 2, you can find the code in the “husk.h” section under the voice “mode 3”. Here I’m going to illustrate the working a bit. The first step is setting the robot right:
digitalWrite(39,HIGH);
digitalWrite(25,HIGH); // turn on Huskylens
Serial1.print(F("HUSKY_START$"));
if (70 <= distance <= 75){
Stop();
switch2 = 1;
Serial.println(F("switch 1"));
Serial1.print(F("sw1$")); //set the robot between 70-75 cm to the target
if((huskylens.request())&&(huskylens.isLearned())&&(huskylens.available())){
HUSKYLENSResult result = huskylens.read();
printResult(result);
if(xCenter>170){
Serial.println(F("←"));
left();
}
else if(xCenter<150){
Serial.println(F("→"));
right();
}
else {
Stop();
Serial.println(F("switch 2"));
Serial1.print(F("sw2$"));
switch2 = 2;
}
}
// center the target
Second step is to evaluate the height and length of the target compared to the robot. For the length the robot uses the ultrasonic sensor mounted on the top of it. Things become a bit more difficult for the height. Here I have to use the Huskylens, but as output data the robot can only receive the position in pixels (px) So I assumed that in a specific range (between 70 and 50 cm) the output data of the ordinate of the point on the Huskylens display has some sort of proportionality with the real height. After I made these assumptions, I empirically found this proportionality. First, I measured some output data in order to evaluate the constant of proportionality (k):
After taking some data (first three columns on the graph), I thought of a way to find k. The major problem was that I have to work with three-dimension points. To make things a little bit easier, I use as ordinates of the points the ratio between height and output data (h and yCenter on the graph). Then I compare this new roast of numbers with the respective distance (d). Once finished I end up with a quite good intersection of the points:
The function that draw this intersection is:
Remembering that y is equal to h/yOrigin with a bit of math I can calculate the height of the target:
At this point, I can use properties’ of right triangle to evaluate the angle of the blaster:
Now α can be easily found by knowing that tan α is equal to the ratio of the height (h) and the distance (d). Once I found tan α, I can calculate α with inverse function:
That in the code ends up in:
float high = ((1.52E-3*distance+0.243) * yCenter) + 5 ;
float α= height/ distance;
float deg = atan(α) / 0.0175;
[husky.h section, lines 127-129]
Note: In the first line a +5 has been added with respect to the equation above. This is an error found empirically , caused by different variables (accuracy of the servo, accuracy of the blaster, calculus approximation of the Arduino).
After this the blaster is set in the right position a shot is taken:
int servoDeg = 90 + int(deg);
servo1.write(servoDeg);
Serial.print(F("servo Deg"));
Serial.println(servoDeg);
Serial.print(F("yCenter: "));
Serial.println(yCenter);
Serial.print(F("distance: "));
Serial.println(distance);
Serial.print(F("high: "));
Serial.println(high);
delay(1500);
shooting();
Serial1.print(F("HUSKY_FINISH$"));
Serial.println(F("finish"));
Serial1.print(F("finish$"));
mode = 1;
}
[husky.h section, lines 131-146]
Obviously, this is a totally ideal situation, made assuming that acceleration of gravity doesn't have any effect on the ball.
This is a video showing this algorithm in action. The red dot is a laser mounted on the blaster. You can follow blaster movements with it.
- Micro:bit code
The code of micro:bit is really simple. Most of his work is to send data, taken by its built-in sensors, to the robot and to another micro:bit. This allows it to reduce sensors on the robot and to have a telemetry system, which can be used to track every second robot’s behaviour.
- Shield
For this project I designed a shield to simplify all the contacts between all the systems. For the design I used the function “electronic design” of fusion. You can find fbrd, fsch and gerber files in the description. I then ordered it on pcbway and soldered all the trough-hole components. The shield contains two ethernet output, six DC motor output, driven by three H-bride, four output for ultrasonic sensors, ? I/O port, an IR sensor, a connection for a LN? and three serial ports.
ACTUATOR SYSTEM
The two main part of this system are the blaster and the gripper. There are also other minor actuators.
- Blaster
The blaster is the most important part of the robot. It works with 15 mm diameter balls printed in 3d. It uses a rubber band system for shooting bullets one by one. It has two 9 grams servomotors that guaranteed a liberty of movement of ±45° horizontally and ±30° vertically. It supports a dispenser mounted on the top part of the chassis that refills the blaster. The blaster block is independent of the chassis and all the robot-blaster connections are made with an ethernet cable. It also includes a laser diode used for pointing the target. For shooting, the blaster has got a DC motor with high ratio. For converting rotary motion to linear a slider-crank mechanism is used. I take the idea for this system by this video of Technic Bricks. The piston is attached to a rubber in order to increase the initial speed of the bullet, following Hooke’s law. Here is a video of how the shooting system works:
- Gripper
The gripper is a classic gripper. In order to be driven, a servo motor is attached at the top of the mechanism. The servo is light (it’s a normal 9 grams servo motor), but it deals perfectly fine with his assignment, in fact the gripper was developed to grab 10x5x5 boxes. This is a video of the gripper in action:
SENSOR SYSTEM
Overall view
There are also a bunch of sensors mounted on the robot. There are two groups of sensors: built-in sensors and external sensors. The built-in category includes all the sensors mounted on the two boards. The majority of them are mounted on the micro:bit. Built-in sensors used in these projects are: temperature and light sensor, accelerometer and compass. External sensors are Huskylens, ultrasonic sensor, ir sensor and a radio sensor.
- Built-in sensors
Built-in sensors are used to send data to telemetry and to Arduino. Temperature sensor is used to increase the accuracy of the ultrasonic sensor. In fact, the speed of the sound changes in relation to temperature, in according to this equation:
That in the code turns out being:
int ultraSens(const int trigPin, const int echoPin){
digitalWrite(48, HIGH);
delayMicroseconds(10);
digitalWrite(48, LOW);
long t = pulseIn(49, HIGH);
tempRequest();
float vs = 0.03314 + 0.000062 * temp;
float d = vs * t / 2;
return d;
}
[sensor.h section, lines 45-55], (the speed of sound equation is underlined)
tempRequest() is a function that read and return the temprature of the micro:bit sensor:
void tempRequest(){
Serial1.print("TEMP_acces$");
Serial1.print("");
if (Serial1.available()){
str = Serial1.readStringUntil('$');
temp = atoi(str.c_str());
}
}
[serialController.h section, lines 59-66]
- Ultrasonic sensor
The ultrasonic sensor is used to measure the distance between the robot and the wall. It is used by the arduino to protect the robot by crashing against the wall. In fact, the robot is able to stop himself at a distance of 10 cm. This algorithm also moves the robot backward up to a distance of 45 cm.
- Huskylens
This sensor is used in the algorithms shown above (Control system, “code” section)
CHASSIS
The fusion file and stl files are in the description. STL files are divided by category, as well as bodies in the STL file. The assembly is really simple. For assembly the frame to assemble the frame, hot glue the inner piece with the two outer ones as shown in the image:
The yellow body is the inner part, gray ones are external pieces.
PCB
Gerber and other files are in the description. There are also BOM file and a PDF with all the connections (PCB_pins).
A robomaster-base robot
*PCBWay community is a sharing platform. We are not responsible for any design issues and parameter issues (board thickness, surface finish, etc.) you choose.
- Comments(2)
- Likes(0)
- 1 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
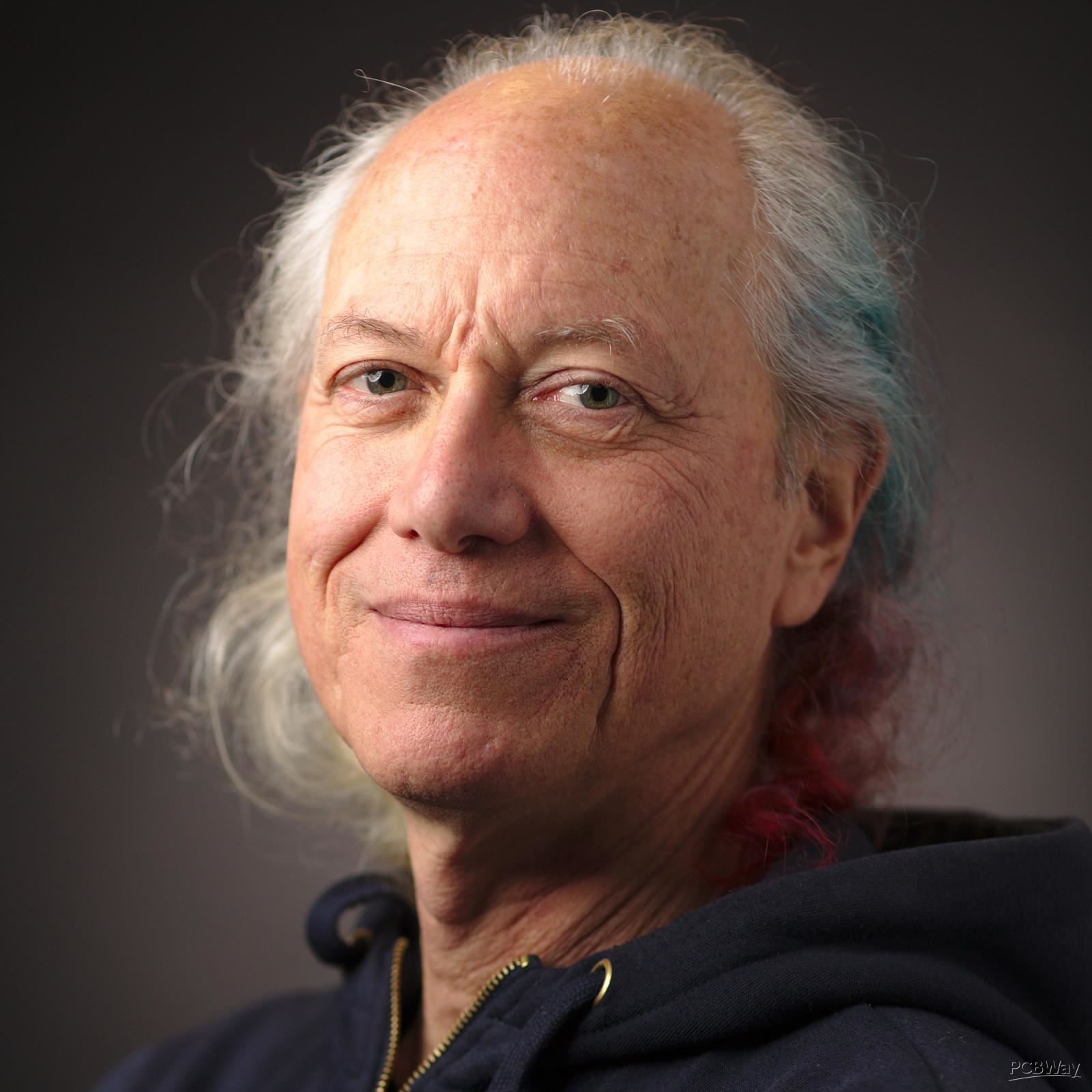
-
8design
-
7usability
-
8creativity
-
7content
More by Antonio Antonio G.
-
-
Micro Planck - 3D Printable Low Profile Mechanical Keyboard
162 1 0 -
Corazon LED intermitente
54 0 0 -
-